Some way to get this automatically:
#(define (Beat_counter_engraver context)
(let ((measpos #f) (grouping #f) (basemom #f) (beatmom #f))
(define (mom->dur mom)
(let ((pair (moment->fraction mom)))
(/ (car pair) (cdr pair))))
(define (is-beat-moment? count gping beatcount)
(if (= (* count (mom->dur basemom)) (mom->dur measpos))
(+ 1 beatcount)
(if (> (* count (mom->dur basemom)) (mom->dur measpos))
#f
(if (null? gping)
#f
(is-beat-moment? (+ count (car gping)) (cdr gping) (+ 1 beatcount))))))
(make-engraver
((process-music engraver)
(set! measpos (ly:context-property context 'measurePosition 'noof))
(set! grouping (ly:context-property context 'beatStructure 'noof))
(set! basemom (ly:context-property context 'baseMoment 'noof))
(set! beatmom (is-beat-moment? 0 grouping 0))
(if beatmom
(let ((grob (ly:engraver-make-grob engraver 'TextScript '())))
(ly:grob-set-property! grob 'text (number->string beatmom))
(ly:grob-set-property! grob 'direction DOWN)
(ly:grob-set-property! grob 'padding 3)))))))
\layout {
\context {
\RhythmicStaff
\consists #Beat_counter_engraver
}
}
\new RhythmicStaff
{
\set RhythmicStaff.baseMoment = #(ly:make-moment 1 16) % manually make sure base moment is 1/16
\set RhythmicStaff.beatStructure = 3,3,3,3 % manually make sure that beats always have 3 16th
\time 6/16
16 16 16 16 16 16|
r16 16 16 8.
\time 3/16
16[ \once\set stemRightBeamCount = 1
\once\set stemLeftBeamCount = 1
r16 16]
\time 6/16
16 16 r r8. \bar "|."
}
%% with different moments:
\new RhythmicStaff {
\time 2/4
8 16 16 8 16 16
\time 3/8
16 16 16 16 16 16
\time 6/8
8 16 16 8 16 16 16 16 16 16
\time 9/7 \tuplet 7/8 { 8 8 8 8 8 8 8 8 8 }
}
which results in
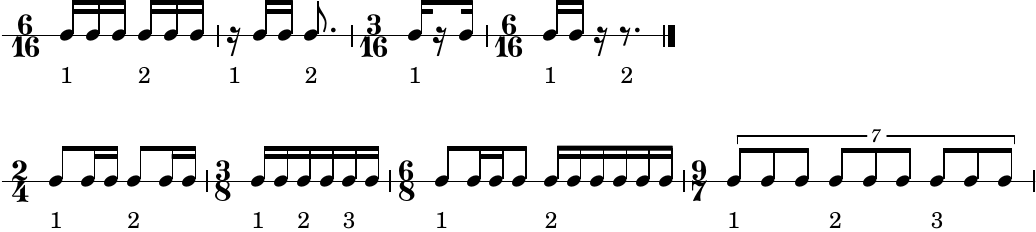
One issue here is that this only works when these is some event on the beat, so if you have something like \time 4/4 8 4 8 this will not output anything on the second beat, since there is nothing there. So you might want to do something like
<< \music \repeat unfold #(floor (/ (/ (car (moment->fraction (ly:music-length music))) (cdr (moment->fraction (ly:music-length music)))) 1/16)) s16 >>
EDIT:
To achieve something like your example you can also do something like this:
#(define (Beat_counter_engraver context)
(let ((measpos #f) (grouping #f) (basemom #f) (beatmom #f))
(define (mom->dur mom)
(let ((pair (moment->fraction mom)))
(/ (car pair) (cdr pair))))
(define (is-beat-moment? count gping beatcount)
(if (= (* count (mom->dur basemom)) (mom->dur measpos))
(+ 1 beatcount)
(if (> (* count (mom->dur basemom)) (mom->dur measpos))
#f
(if (null? gping)
#f
(is-beat-moment? (+ count (car gping)) (cdr gping) (+ 1 beatcount))))))
(make-engraver
((process-music engraver)
(set! measpos (ly:context-property context 'measurePosition 'noof))
(set! grouping (ly:context-property context 'beatStructure 'noof))
(set! basemom (ly:context-property context 'baseMoment 'noof))
(set! beatmom (is-beat-moment? 0 grouping 0))
(if beatmom
(let ((grob (ly:engraver-make-grob engraver 'TextScript '())))
(ly:grob-set-property! grob 'text (number->string beatmom))
(ly:grob-set-property! grob 'direction DOWN)))))))
\layout {
\context {
\Dynamics
\consists #Beat_counter_engraver
\override TextScript.font-shape = ##f
\override TextScript.Y-offset = #-0.8
\consists Instrument_name_engraver
}
}
sixteenth-grid-during =
#(define-music-function (m) (ly:music?)
#{
\repeat unfold #(floor (/ (/ (car (moment->fraction (ly:music-length m))) (cdr (moment->fraction (ly:music-length m)))) 1/16)) s16
#})
\paper {
ragged-last = ##f
}
musI = {
\mark "In 3"
\time 3/8
8 8 16 r |
8 16 16 8 |
16[ 16 8 r16 16] |
4. \bar "|."
}
>
musII = {
\mark "In 4"
\time 4/8
\set Score.beatStructure = 1,1,1,1
8 16 16~ 8 r8 |
r16 16[ r16 16] r16 8.~ |
4 ~ 16 8 16 |
16 r16 4 r8 \bar "|."
}
>
which gives you
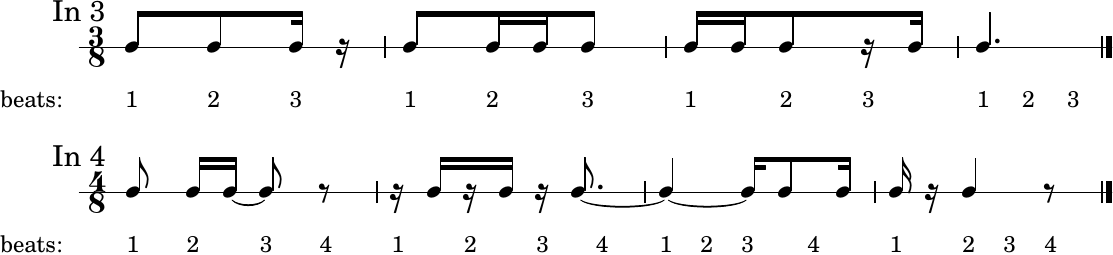